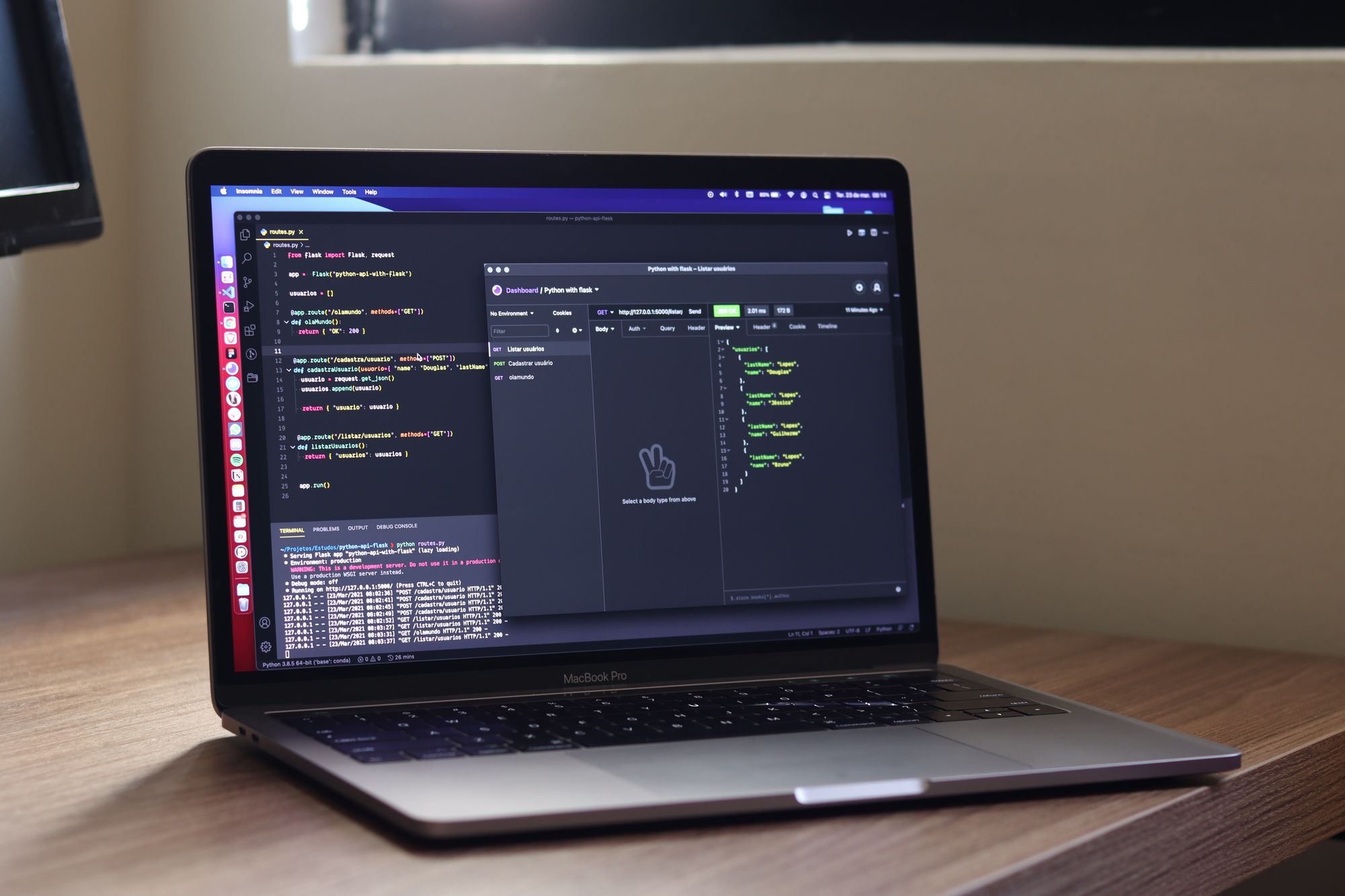
Recently, Onfleet has released the PHP API wrapper that offers ways to make calls to Onfleet’s API with very little engineering effort. Onfleet’s PHP API wrapper is a robust, fully tested set of easy-to-use functions that help developers build integrations with Onfleet fast. The source code and the documentation can be found on Github and Packagist.
In order to use the PHP API wrapper, you will need to have a webserver running PHP 7.4 or higher, a project that is using composer, and a bcmath extension enabled.
Once the server and the project are in place, it is time to install the PHP wrapper package. It can be easily done by running:
composer require onfleet/php-onfleet
When the package is installed we need to make sure that files are autoloading. If you do not have any other autoloading logic in place you can use:
require_once "vendor/autoload.php";
Next, you need to include Onfleet’s namespace:
use Onfleet\Onfleet;
Now, you will need an API key. To set up your API key please follow this quick guide.
Here is an example of the basic index.php file to test how things are loading and working:
<?php
require_once "vendor/autoload.php";
use Onfleet\Onfleet;
$api = new Onfleet("86847*****************", 6000);
echo $api->verifyKey();
Now let’s try to create a task. Let us use an example shared by my colleague Mariana in her article “Creating your first Onfleet task via the API”. Let’s say we run a donut shop and have just received an order with the following information:
- Customer: Bruce Wayne
- Phone Number: +1-905-555-5555
- Address: 30 Rockefeller Plaza; New York, NY 10112
- Complete After: February 27, 2022
- Order Notes: 1 dozen assorted donuts
The code to create a task with this information is:
$data = [
"destination" => [
"address" => [
"unparsed" => "30 Rockefeller Plaza; New York, NY 10112",
],
"notes" => ""
],
"recipients" => [
[
"name" => "Bruce Wayne",
"phone" => "+1-905-555-5555",
"notes" => ""
]
],
"completeAfter" => 1645912800000,
"notes" => "1 dozen assorted donuts"
];
$response = $api->tasks->create($data);
Note that we are still using the `$api` variable from the example shared above.
This is the response we get from the API:
Array(
[id] => 4kK43QYz~ByrXu61InjqxNTp
[timeCreated] => 1644511668000
[timeLastModified] => 1644511668532
[organization] => RYjeROPOSae0L5lnZr6rlcRy
[shortId] => 9619dcc7
[trackingURL] => https://onf.lt/9619dcc7fa
[worker] =>
[merchant] => RYjeROPOSae0L5lnZr6rlcRy
[executor] => RYjeROPOSae0L5lnZr6rlcRy
[creator] => zbWWnWCN7l3ypC9gipRXvpH~
[dependencies] => Array()
[state] => 0
[completeAfter] => 1645912800000
[completeBefore] =>
[pickupTask] =>
[notes] => 1 dozen assorted donuts
[completionDetails] => Array(
[failureNotes] =>
[failureReason] => NONE
[events] => Array()
[actions] => Array()
[time] =>
[firstLocation] => Array()
[lastLocation] => Array()
[unavailableAttachments] => Array()
)
[feedback] => Array()
[metadata] => Array()
[overrides] => Array(
[recipientName] =>
[recipientNotes] =>
[recipientSkipSMSNotifications] =>
[useMerchantForProxy] =>
)
[quantity] => 0
[additionalQuantities] => Array
(
[quantityA] => 0
[quantityB] => 0
[quantityC] => 0
)
[serviceTime] => 0
[identity] => Array(
[failedScanCount] => 0
[checksum] =>
)
[appearance] => Array(
[triangleColor] =>
)
[scanOnlyRequiredBarcodes] =>
[container] => Array(
[type] => ORGANIZATION
[organization] => RYjeROPOSae0L5lnZr6rlcRy
)
[trackingViewed] =>
[recipients] => Array(
[0] => Array(
[id] => 09Z~GJe7UaUTgAlXnmdiS25t
[timeCreated] => 1618248279000
[timeLastModified] => 1644511668457
[name] => Bruce Wayne
[phone] => +19055555555
[notes] =>
[organization] => RYjeROPOSae0L5lnZr6rlcRy
[skipSMSNotifications] =>
[metadata] => Array()
)
)
[destination] => Array(
[id] => 42SHTjCUZZEGGYMS7QRKjM*9
[timeCreated] => 1644511668000
[timeLastModified] => 1644511668434
[location] => Array(
[0] => -73.9799726
[1] => 40.7593755
)
[address] => Array(
[apartment] =>
[state] => New York
[postalCode] => 10112
[city] => New York
[country] => United States
[name] => Rockefeller Plaza
)
[notes] =>
[metadata] => Array()
[googlePlaceId] => ChIJ4xM00f5YwokRLqBArQncYlw
[warnings] => Array()
)
)
Now let’s say we want to assign this task to a driver but we do not have a driver yet. We can create a driver with the following code:
$workerData = [
"name" => "A Swartz",
"phone" =>"617-342-8853",
"teams" => ["vgjtQU6tJQ4wsiBZwgStO5M2"],
"vehicle" => [ "type" => "CAR",
"description" => "Tesla Model S",
"licensePlate" => "FKNS9A",
"color" => "purple" ]
];
$worker = $api->workers->create($workerData);
Note that I added the team, the id of the team can be stored at team creation with a call to create-team or queried via the API in a list-teams call.
So the driver created looks like this:
Array(
[id] => 40kMWRSQVtb8PJZsMP*cZvz0
[timeCreated] => 1644576124000
[timeLastModified] => 1644576124337
[organization] => RYjeROPOSae0L5lnZr6rlcRy
[name] => A Swartz
[displayName] =>
[phone] => +16173428853
[activeTask] =>
[tasks] => Array()
[onDuty] =>
[timeLastSeen] =>
[capacity] => 0
[additionalCapacities] => Array(
[capacityA] => 0
[capacityB] => 0
[capacityC] => 0
)
[userData] => Array()
[accountStatus] => INVITED
[metadata] => Array()
[timezone] =>
[imageUrl] =>
[teams] => Array(
[0] => vgjtQU6tJQ4wsiBZwgStO5M2
)
[addresses] => Array(
[routing] =>
)
[vehicle] => Array(
[id] => OjnajtAK3AKGZHlILQl~6E2V
[type] => CAR
[description] => Tesla Model S
[licensePlate] => FKNS9A
[color] => purple
[timeLastModified] => 1644576124323
)
)
Well, this driver cannot take tasks yet as he was just invited to the app and he/she will need to install the app, create an account and set a permanent password. I do have a team created before and I have its ID, we can auto-assign a task to a driver within that team. Here is how to do it:
$data = [
"tasks" => ["4kK43QYz~ByrXu61InjqxNTp"],
"options" => [
"mode" => "distance",
"team" => "vgjtQU6tJQ4wsiBZwgStO5M2"
]
];
$response = $api->tasks->autoAssign($data);
Here we can see that the task was assigned to a driver within the specified team:
Array(
[assignedTasksCount] => 1
[assignedTasks] => Array(
[4kK43QYz~ByrXu61InjqxNTp] => vcLDzF8ARDPJRL1MYueuV928
)
)
So now donuts will be delivered to Bruce Wayne and you know how to work with Onfleet’s API using the PHP API wrapper.
Should you have any questions in terms of using the PHP API wrapper or interacting with Onfleet’s API, please reach out to Support at support@onfleet.com.